Parallel Processing with Rayon: Optimizing Rust for the Multi-Core Era
Learn how to use the Rayon library in Rust for parallel programming. Explore core concepts, key features, practical examples, and best practices to enhance performance with multi-core processors.

For many years, Moore's Law—the observation that transistor density doubles approximately every two years—drove advancements in processor technology. This, along with improved architectures and faster clock speeds, led to substantial performance gains in single-core processors. However, we're now encountering physical constraints in chip manufacturing, resulting in a deceleration of single-core performance improvements.
This paradigm shift has far-reaching consequences for software development. As the benefits from faster individual cores wane, the industry has pivoted towards multi-core architectures to push performance boundaries. The future of high-performance computing is increasingly focused on efficiently leveraging multiple cores in parallel rather than relying solely on faster individual cores.
This transition necessitates a shift towards parallel programming techniques to achieve peak performance for software developers. By spreading computational workloads across multiple cores, we can dramatically boost our applications' speed and efficiency. However, effective parallel programming comes with challenges, including thread management, resource coordination, and avoiding common pitfalls like race conditions and deadlocks.
Rayon is a powerful library for Rust that addresses these challenges. Rayon provides robust tools for parallel computation while preserving Rust's renowned safety guarantees. It offers a high-level abstraction for data parallelism, simplifying the process of writing concurrent code. Often, developers can parallelize existing sequential code with minimal modifications.
In this article, we'll look at Rayon and explore how it enables efficient, parallel programming in Rust for today's multi-core systems. We'll examine core concepts, highlight key features, walk through practical examples, and discuss best practices.
The Challenge of Parallelism
Modern hardware typically features multi-core processors, yet many applications still run predominantly on a single core, leaving substantial computing power untapped. Parallel processing aims to harness this potential by dividing computational tasks into smaller units that can execute simultaneously across multiple cores. This approach can dramatically accelerate performance, especially for computationally intensive tasks and large datasets, while maximizing the use of available hardware resources.
However, writing effective parallel code presents significant challenges. Developers must carefully manage threads, coordinate access to shared resources, and navigate complex pitfalls like race conditions and deadlocks. These difficulties have traditionally made parallel programming a specialized skill, often reserved for performance-critical sections of code.
Enter Rayon: Rust's Solution for Easy Parallelism
With its focus on performance and safety, Rust provides a solid foundation for parallel programming. The Rayon library builds on this, offering a high-level abstraction for data parallelism that simplifies writing concurrent code.
Critical aspects of Rayon include:
- An intuitive API that often enables parallelization with minimal code changes
- Automatic work-stealing to balance load across available cores
- Guaranteed data-race freedom, leveraging Rust's ownership system
With Rayon, developers can often parallelize existing sequential code by changing iter()
to par_iter()
. This simplicity, combined with Rust's performance characteristics, makes Rayon a powerful tool for optimizing computationally intensive tasks.
Exploring Rayon
This post will dive into the practical aspects of using Rayon in Rust projects. We'll cover:
- Core concepts and usage patterns
- Key parallel algorithms provided by Rayon
- Advanced features for fine-tuning parallel execution
- Best practices and common pitfalls
- How to integrate Rayon into a larger project
Let's explore how Rayon can help us write efficient, parallel Rust code for the multi-core era.
What is Rayon?
Rayon is a data-parallelism library for Rust. At its core, it's designed to make it easy to convert sequential computations into parallel ones. Developed by Niko Matsakis and Josh Stone, Rayon has become a cornerstone of parallel programming in the Rust ecosystem.
The name "Rayon" is a play on words—it's a type of fibre known for its strength when woven together, much like how Rayon weaves together parallel computations for increased performance.
How Rayon Simplifies Parallel Programming in Rust
Rayon's primary goal is to make parallel programming accessible and safe. It achieves this through several fundamental design principles:
- Minimal API Changes: Often, you can parallelize existing code by changing just a single method call. For example, changing
iter()
topar_iter()
can transform a sequential operation into a parallel one. - Work Stealing: Rayon uses a work-stealing algorithm to balance the computational load across available CPU cores efficiently. You don't need to divide work or manage thread pools manually.
- Data Race Prevention: By leveraging Rust's ownership and borrowing rules, Rayon ensures that parallel code is free from data races by default.
- Composability: Parallel iterators in Rayon can be composed just like regular iterators, allowing for complex parallel computations to be built from simple components.
Here's a simple example to illustrate how easy it is to use Rayon:
use rayon::prelude::*;
fn sum_of_squares(input: &[i32]) -> i32 {
input.par_iter() // This is the only change needed
.map(|&i| i * i)
.sum()
}
In this code, changing iter()
to par_iter()
parallelizes the entire computation.However, it's crucial to understand that this simplicity doesn't guarantee performance improvements. Let's look at some benchmark results:
Array Size | Sequential (µs) | Parallel (µs) | Parallel Efficiency |
---|---|---|---|
100 | 0.00627 | 15.101 | 0.00042 |
1,000 | 0.04197 | 20.947 | 0.00200 |
10,000 | 0.53719 | 29.714 | 0.01808 |
100,000 | 5.5541 | 40.420 | 0.13741 |
1,000,000 | 55.739 | 72.152 | 0.77252 |
These results highlight several important points:
- For this simple operation (squaring and summing integers), the sequential version outperforms the parallel version across all tested array sizes.
- The overhead of parallelization is significant for smaller array sizes. This includes the cost of creating and managing threads, as well as the work-stealing algorithm.
- As the array size increases, the performance gap narrows, but even for 1 million elements, the sequential version is still faster.
- The simplicity of Rayon's API doesn't guarantee performance improvements. It's crucial to benchmark your specific use case to determine if parallelization is beneficial.
- Rayon's benefits are more likely to be seen with more complex operations or larger datasets than those tested here.
These results underscore the importance of understanding your workload and benchmarking your specific use case. While Rayon makes it easy to write parallel code, the performance benefits depend on factors such as the complexity of the operation, the size of the dataset, and the characteristics of the hardware.
In practice, Rayon tends to shine with more computationally intensive tasks or when working with very large datasets. For simple operations like integer arithmetic, especially on smaller datasets, the overhead of parallelization can outweigh the benefits.
Key Features and Advantages
- Par-Iter API: Rayon provides parallel versions of many iterator methods (
map
,filter
,reduce
, etc.), allowing for easy parallelization of existing iterator chains. - Join Operation: For more complex parallel algorithms, Rayon offers a
join
function that splits computations into two parallel tasks. - Custom Thread Pools: While Rayon's global thread pool works well for most cases, you can create custom thread pools for more fine-grained control.
- Scoped Threads: Rayon allows for creating scoped thread pools, enabling the use of references to stack data in parallel computations.
- Parallel Collection Types: Rayon includes parallel versions of common collections like
Vec
andHashMap
, allowing for efficient parallel operations on these data structures. - Adaptive Parallelism: Rayon automatically adjusts the degree of parallelism based on available system resources and the size of the problem.
- Nested Parallelism: Rayon efficiently handles nested parallel computations, automatically managing thread allocation to prevent oversubscription.
The key advantage of Rayon is that it allows developers to write parallel code nearly as simple as sequential code while still achieving significant performance improvements on multi-core systems. It does this without sacrificing Rust's strong safety guarantees, making it a powerful tool for building efficient, concurrent software.
Getting Started with Rayon
Now that we understand what Rayon is and its key features let's dive into how to start using it in your Rust projects.
Adding Rayon to Your Rust Project
First, add Rayon as a dependency in your Cargo.toml
file:
[dependencies]
rayon = "1.10.0"
image = "0.25.1"
We're also adding the image
crate for our example.
Real-World Example: Parallel Image Processing
Let's implement a parallel image processing task: applying a blur effect to an image. This example demonstrates Rayon's power in a computationally intensive scenario.
use image::{ImageBuffer, Rgb};
use rayon::prelude::*;
use std::env;
fn parallel_blur(
img: &ImageBuffer<Rgb<u8>, Vec<u8>>,
blur_radius: u32,
) -> ImageBuffer<Rgb<u8>, Vec<u8>> {
let (width, height) = img.dimensions();
let mut output: ImageBuffer<Rgb<u8>, Vec<u8>> = ImageBuffer::new(width, height);
output
.enumerate_pixels_mut()
.par_bridge()
.for_each(|(x, y, pixel)| {
let mut r_total = 0;
let mut g_total = 0;
let mut b_total = 0;
let mut count = 0;
for dy in -(blur_radius as i32)..=(blur_radius as i32) {
for dx in -(blur_radius as i32)..=(blur_radius as i32) {
let nx = x as i32 + dx;
let ny = y as i32 + dy;
if nx >= 0 && nx < width as i32 && ny >= 0 && ny < height as i32 {
let p = img.get_pixel(nx as u32, ny as u32);
r_total += p[0] as u32;
g_total += p[1] as u32;
b_total += p[2] as u32;
count += 1;
}
}
}
pixel[0] = (r_total / count) as u8;
pixel[1] = (g_total / count) as u8;
pixel[2] = (b_total / count) as u8;
});
output
}
fn main() -> Result<(), image::ImageError> {
let current_dir = env::current_dir()?;
println!("Current working directory: {:?}", current_dir);
let img = image::open("input.jpg")?.to_rgb8();
let blur_radius = 15;
let blurred = parallel_blur(&img, blur_radius);
blurred.save("output.jpg")?;
Ok(())
}
This is suboptimal example code
This example demonstrates several key Rayon concepts:
- Parallel Iteration: We use
par_bridge()
to create a parallel iterator from the sequentialenumerate_pixels_mut()
iterator. - Data Parallelism: Each pixel's blur calculation is independent, making this task ideal for parallelization.
- Work Division: Rayon automatically divides the work across available CPU cores.
- Shared Immutable State: The input image is shared immutably across all parallel tasks.
- Mutable Output: We're directly modifying the output buffer in parallel.
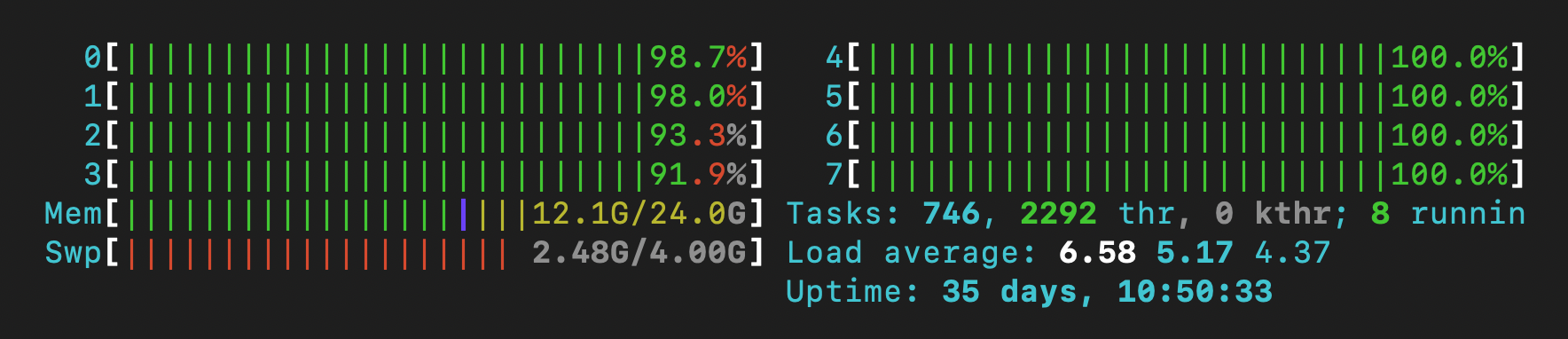
Key points in this implementation:
enumerate_pixels_mut()
: This method from theimage
crate gives us an iterator over mutable pixel references along with their coordinates.par_bridge()
: This Rayon method converts a regular iterator into a parallel iterator, allowing us to use Rayon's parallel processing capabilities.- The blur algorithm calculates each pixel's new value by averaging the values of surrounding pixels within the specified radius.
This example showcases Rayon's ability to simplify parallel programming in Rust. We're performing a computationally intensive task (blurring an image) in parallel with relatively straightforward code. Rayon handles the complexities of work distribution and thread management, allowing us to focus on the algorithm itself.
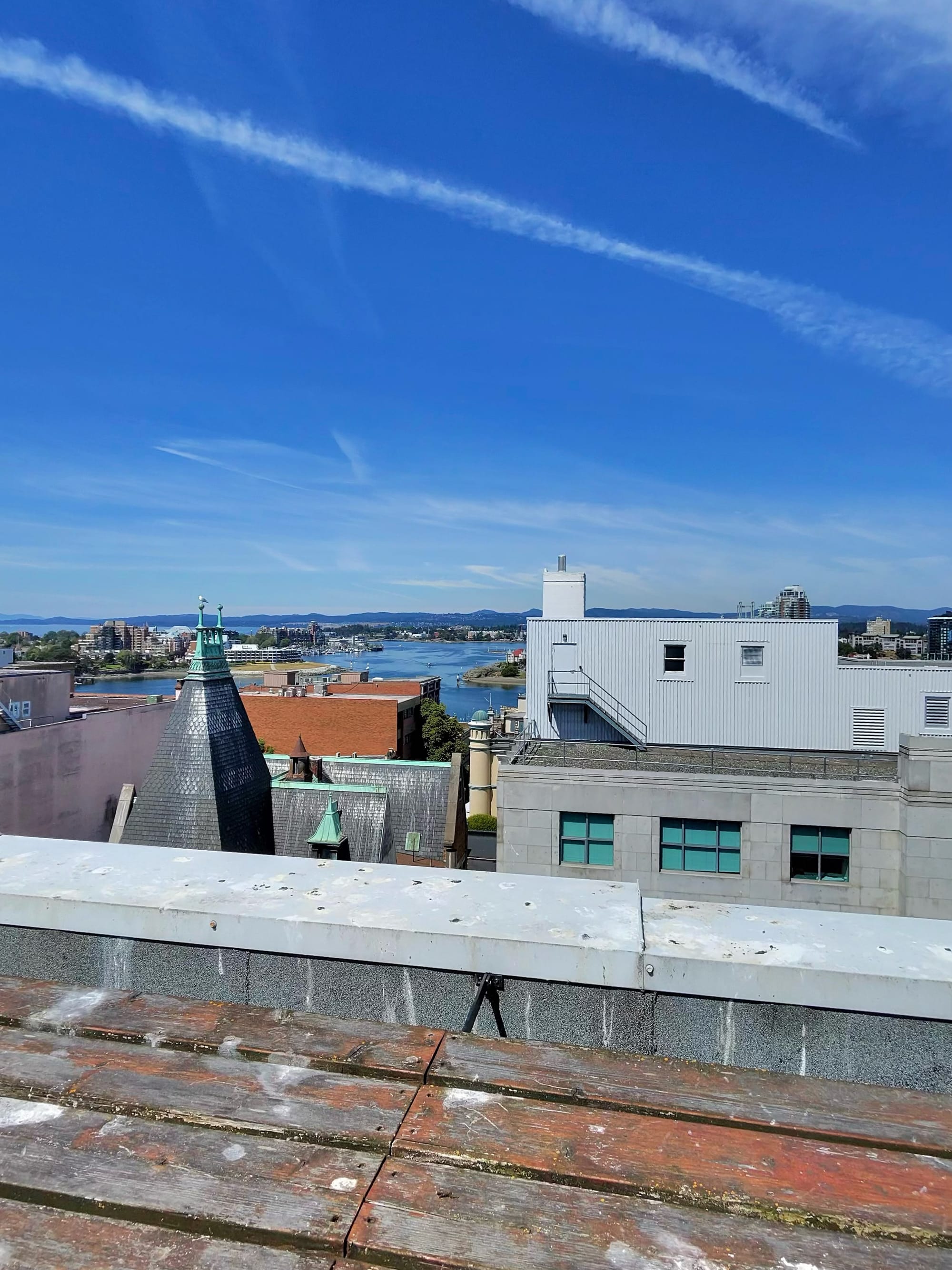
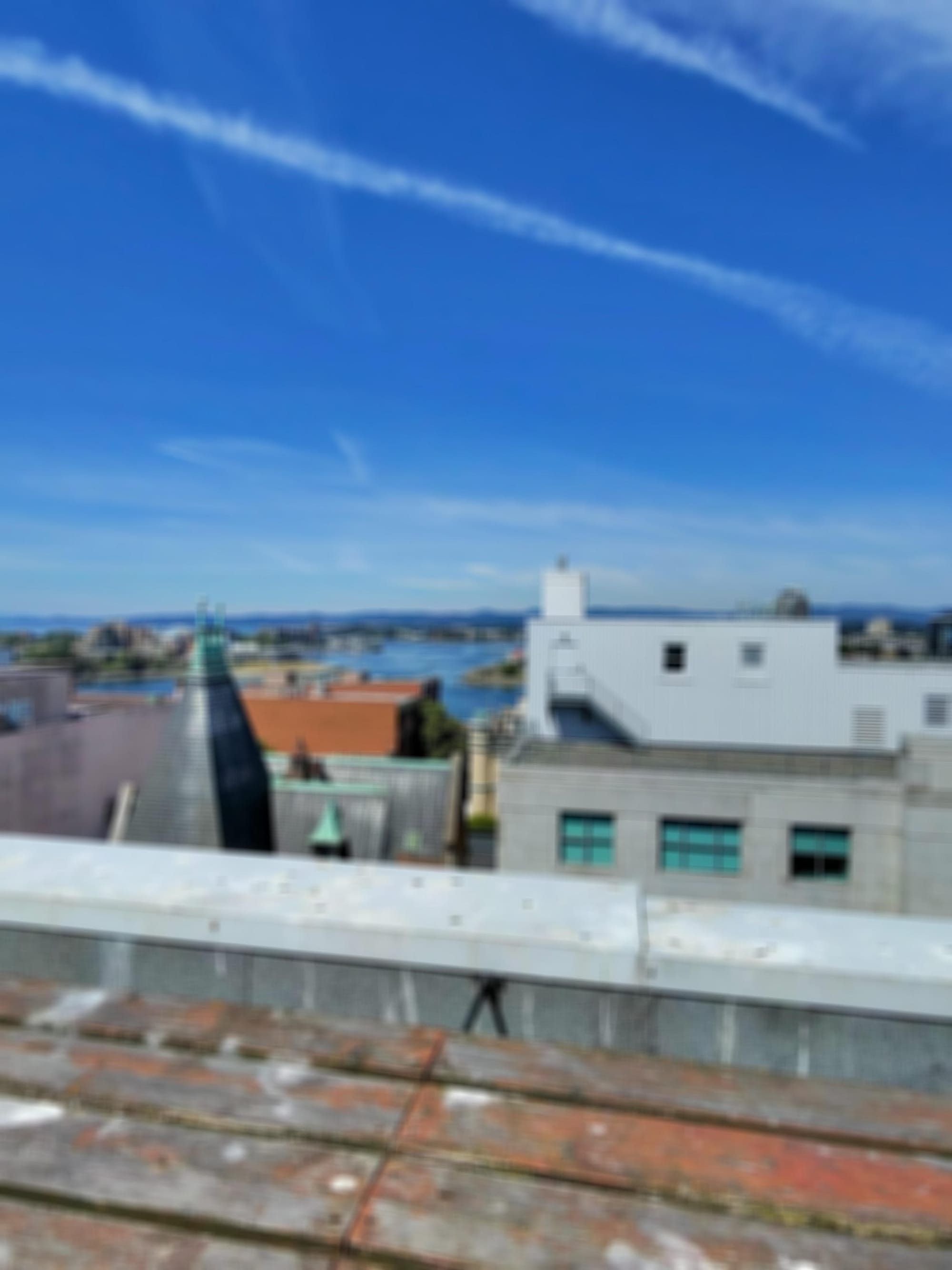
Before and after blurring
In the following sections, we'll explore more advanced Rayon features and best practices for writing efficient parallel code.
Best Practices and Considerations
While Rayon simplifies parallel programming in Rust, it's crucial to understand when and how to use it effectively. Let's explore some best practices and important considerations.
When to Use Parallelism (and When Not To)
Parallelism can significantly improve performance, but it's not always the right solution. Here are some guidelines:
Use parallelism when:
- You have computationally intensive tasks that can be divided into independent units of work.
- Your data set is large enough that the benefits of parallel processing outweigh the overhead of thread management.
- You're working with CPU-bound tasks rather than I/O-bound tasks.
Avoid parallelism when:
- Your tasks are too small or simple. The overhead of creating and managing threads might exceed the performance gain.
- Your operations are primarily I/O bound. In these cases, asynchronous programming might be more appropriate.
- You have complex dependencies between tasks that require frequent synchronization.
Remember, the key to effective parallelism is having enough work to distribute across cores. Our image blurring function is a good candidate because each pixel calculation is independent and computationally intensive. In contrast, summing a small array of integers might be faster sequentially due to the overhead of parallel execution.
Balancing Work Across Threads
Rayon aims to balance work automatically, but you can help it perform better:
- Chunk size considerations: For operations on large collections, processing data in larger blocks can reduce overhead. Rayon provides methods like
par_chunks()
orpar_chunks_mut()
for this purpose. - Avoid unpredictable workloads: Try to ensure that each parallel task has roughly the same amount of work. Highly variable workloads can lead to inefficient thread utilization.
- Use
join
for recursive algorithms: For divide-and-conquer algorithms, Rayon'sjoin
function can be more efficient than using parallel iterators. This is particularly useful for problems like parallel quicksort or tree traversals. - Be mindful of task granularity: Creating too many small tasks can overwhelm Rayon's work-stealing scheduler and lead to poor performance. For recursive algorithms, consider using a sequential approach for small inputs.
Here's an example of how not to use join
:
fn inefficient_parallel_fibonacci(n: u64) -> u64 {
if n <= 1 {
return n;
}
let (a, b) = rayon::join(
|| inefficient_parallel_fibonacci(n - 1),
|| inefficient_parallel_fibonacci(n - 2),
);
a + b
}
This implementation creates an exponential number of tiny tasks, leading to extremely poor performance for large n. Instead, consider a hybrid approach that switches to sequential computation below a certain threshold, or restructure your algorithm to use parallel iterators on larger chunks of work.
The goal is to provide enough work for each thread to do, minimizing the overhead of task distribution and maximizing CPU utilization.
Ensuring Correctness in Parallel Code
Rust's ownership system and borrowing rules prevent data races at compile time, which is a significant advantage when writing parallel code. However, there are still important considerations when using Rayon:
- Understanding Rayon's execution model: Rayon uses a work-stealing scheduler, which means the order of execution is not guaranteed. Don't rely on any specific execution order in parallel code. This is particularly important when you're converting sequential code to parallel and might be making assumptions about ordering.
- Avoiding logical races: While Rust prevents data races, logical races (where the outcome depends on the order of operations) can still occur. Be mindful of operations that depend on ordering, especially when using methods like
for_each
orreduce
. - Proper use of synchronization primitives: When you do need to share mutable state across threads, use appropriate synchronization primitives like
Mutex
or atomic types. Rust ensures you use these correctly, but it's still important to understand their performance implications. - Careful use of early returns: Using
return
,break
, orcontinue
inside a parallel iterator can lead to unexpected behavior. Instead, use Rayon's provided methods likefind_any
orany
for early termination in parallel contexts. - Managing complex lifetimes: When working with borrowed data in parallel contexts, Rayon's
scope
function can help manage lifetimes safely. - Awareness of interior mutability: When using types with interior mutability (like
RefCell
), be aware that the borrow checking happens at runtime. In parallel contexts, prefer thread-safe alternatives likeRwLock
.
Here's an example of using scope
to safely access borrowed data in parallel:
use rayon::prelude::*;
fn main() {
let mut numbers = vec![1, 2, 3, 4, 5];
// Using scope to work with borrowed data in parallel
rayon::scope(|s| {
// Spawn a task that borrows `numbers` immutably
s.spawn(|_| {
// Calculate sum of squares
let sum: i32 = numbers.par_iter().map(|&x| x * x).sum();
println!("Sum of squares: {}", sum);
});
// Spawn another task that borrows `numbers` immutably
s.spawn(|_| {
let doubled: Vec<i32> = numbers.par_iter().map(|&x| x * 2).collect();
println!("Doubled numbers: {:?}", doubled);
});
// Both tasks are guaranteed to complete before the scope ends
});
// After the scope, we can modify `numbers` again
numbers.par_iter_mut().for_each(|x| *x *= 3);
println!("Tripled original numbers: {:?}", numbers);
}
By leveraging Rust's safety guarantees and following these best practices, you can write efficient, correct parallel code with Rayon. Remember, the goal is to maximize parallelism where it makes sense, while ensuring the correctness of your program. Rust's compiler is a powerful ally in this endeavor, but understanding the specific considerations of parallel programming is still crucial.
Integrating Rayon in Larger Projects
As your projects grow in size and complexity, integrating Rayon effectively becomes more challenging but also more rewarding. The key is to understand how Rayon fits into the broader ecosystem of Rust concurrency tools, how to introduce it gradually, and how to ensure your parallel code is correct and performant.
Combining Rayon with Other Rust Concurrency Primitives
Rayon excels at data parallelism, but it's not a one-size-fits-all solution for concurrency. In larger projects, you'll often need to combine Rayon with other concurrency primitives to achieve the best results.
For instance, when working with asynchronous code using Tokio, you might use Rayon for CPU-bound tasks within a Tokio runtime. You can spawn Rayon computations using tokio::task::spawn_blocking
, but be mindful of potential thread pool contention. In some cases, it might be beneficial to use a separate Rayon thread pool to avoid interfering with Tokio's runtime.
Channels, such as those provided by the crossbeam
crate, can be effective for communicating between Rayon-parallelized components. You might implement a producer-consumer pattern where Rayon handles parallel production or consumption of data. Here's an example that demonstrates this:
use crossbeam::channel;
use rayon::prelude::*;
use std::time::Duration;
fn main() {
let (sender, receiver) = channel::bounded(100);
// Producer: Uses Rayon to generate data in parallel
std::thread::spawn(move || {
(0..1000).into_par_iter().for_each(|i| {
let data = expensive_computation(i);
sender.send(data).unwrap();
});
});
// Consumer: Processes the data sequentially
let sum: u64 = receiver.iter().take(1000).sum();
println!("Sum of processed data: {}", sum);
}
fn expensive_computation(i: u64) -> u64 {
// Simulate an expensive computation
std::thread::sleep(Duration::from_millis(1));
i * i
}
This example uses Rayon to parallelize data generation, while using a crossbeam
channel to communicate between the parallel producer and the sequential consumer. This pattern can be useful when you have a computationally intensive task that produces data that needs to be processed or aggregated in a specific order.
For simple shared state in Rayon parallel operations, atomic types from std::sync::atomic
can be very effective. These can be combined with Rayon's reduce
operations for more complex aggregations.
When shared mutable state is unavoidable, you may need to resort to locks like Mutex
or RwLock
. However, in Rayon parallel sections, it's generally better to use coarse-grained locking to minimize contention.
Strategies for Gradually Introducing Parallelism
Introducing parallelism to an existing project should be done incrementally. Start by profiling your application to identify CPU-intensive hotspots. Look for loops or recursive functions operating on large datasets - these are often good candidates for parallelization.
It's usually best to begin parallelizing at the lowest level of your call hierarchy - the leaf functions. This approach minimizes the impact on existing code structure. As you gain confidence, you can gradually expand to higher-level functions.
Consider implementing parallel versions behind feature flags. This allows for easy comparison and fallback to sequential versions. It's crucial to benchmark rigorously, measuring performance before and after parallelization. Be prepared to revert if parallelism doesn't yield improvements - sometimes, the overhead of parallelization can outweigh its benefits for smaller datasets or simpler operations.
As you expand your use of Rayon, you might find opportunities to redesign data structures or algorithms to be more parallelism-friendly. Always document parallel sections of your code clearly, explaining any non-obvious performance characteristics or trade-offs.
Testing Parallel Code
Testing parallel code introduces unique challenges. One of the main issues is non-determinism: Rayon's parallel iterators may execute in different orders on different runs. Design your tests to be order-independent where possible.
Stress testing is crucial for parallel code. Implement tests that run your parallel code many times under varying loads and with different thread counts. This can help uncover subtle threading-related issues.
Property-based testing, using libraries like proptest
, can be very effective for parallel code. Generate diverse inputs for your parallel functions and verify that they produce equivalent results to their sequential counterparts.
Don't forget to include benchmarks in your test suite. These can catch performance regressions that might otherwise go unnoticed. Rust's built-in benchmark tests or libraries like criterion
can be useful here.
Finally, ensure your CI pipeline is configured to run tests on multi-core machines. Consider running tests with different numbers of threads to catch any thread-count-dependent bugs.
By following these strategies, you can effectively integrate Rayon into larger projects, gradually introducing parallelism where it's most beneficial, and ensuring the correctness and performance of your parallel code through comprehensive testing. Remember, the goal is not to parallelize everything, but to apply parallelism judiciously where it provides clear benefits.
Conclusion
As we've explored throughout this post, Rayon stands out as a powerful tool in Rust's ecosystem for parallel computing. Its ability to simplify the complex task of writing parallel code, while leveraging Rust's safety guarantees, makes it an invaluable asset for developers looking to harness the full power of modern multi-core processors.
Rayon's key strength lies in its intuitive API. By providing parallel versions of familiar iterator methods, it allows developers to parallelize their code with minimal changes, often by simply replacing iter()
with par_iter()
. This ease of use, combined with Rayon's work-stealing scheduler, enables efficient utilization of available CPU cores without the need for manual thread management.
Moreover, Rayon's integration with Rust's type system ensures that many common pitfalls of parallel programming, such as data races, are caught at compile-time. This safety-first approach allows developers to write parallel code with confidence, focusing on the logic of their algorithms rather than worrying about low-level concurrency issues.
Looking to the future, parallel processing in Rust is set to become even more crucial. As we approach the physical limits of single-core performance, the ability to effectively utilize multi-core architectures will be key to achieving performance gains. Rust, with its focus on systems programming and performance, is well-positioned to be at the forefront of this parallel computing revolution.
Here are a couple more great resources for further reading:
- The official Rayon documentation provides comprehensive coverage of the library's features and usage patterns.
- Programming Rust by Jim Blandy and Jason Orendorff includes a chapter on parallel programming with Rayon.
- Speed up your Rust code with Rayon by Let's Get Rusty is a helpful video on the topic.
- Data Parallelism with Rust and Rayon by Joshua Mo is a good primer on the topic.
In conclusion, Rayon represents a significant step forward in making parallel programming accessible and safe. As we move into an increasingly parallel future, tools like Rayon will be essential in helping developers write efficient, scalable software that can fully utilize the power of modern hardware.
If you found this interesting, I also wrote an article on using SIMD for parallel processing.
I would like to thank Dr. Stefan Salewski for his generous review of and helpful suggestions for this article.
Discussion